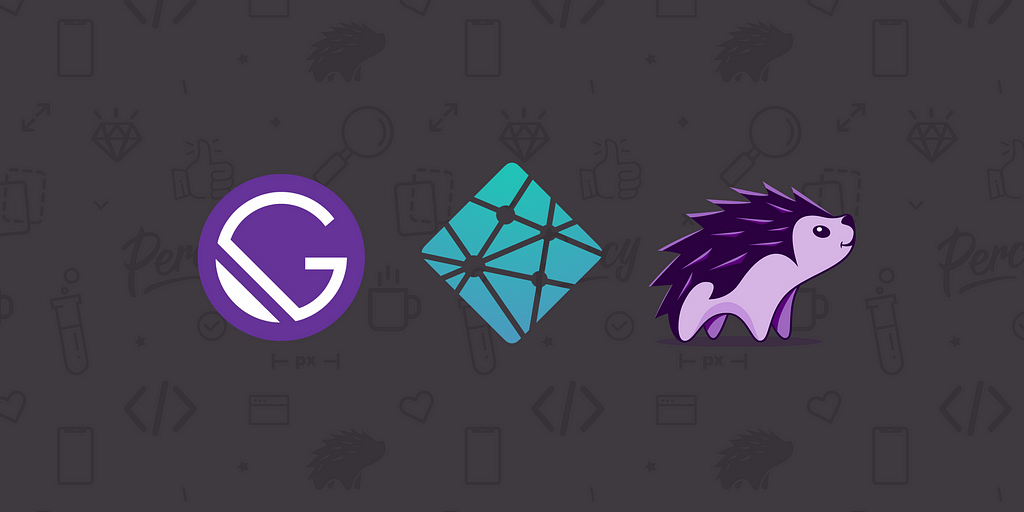
As one of the most popular “static site” generators, the React-based Gatsby has been adopted by thousands of teams to create e-commerce websites, developer blogs, portfolios, and more.
Gatsby is a robust framework that debunks the common misconception that static sites lack complexity or interactability. That’s why testing your Gatsby project is just as important as it is when building anything else — whether with unit testing, component testing, or end-to-end testing.
With Percy’s new Gatsby plugin, it’s now easier than ever to test your Gatsby project from a visual standpoint too.
When running alongside continuous deployments — with Netlify or Gatsby’s newly released Gatsby Builds — Percy can provide teams with seamless and continuous visual testing. It works by generating UI screenshots of your project whenever you build your site and comparing them against baselines to see if anything has changed visually.
In this tutorial, we’ll integrate visual testing into a Gatsby project, set up continuous deployments with Netlify, and do an end-to-end visual review.
We’re going to set up an example Gatsby site, but you can easily adapt this tutorial to work for your own application.
Starting from scratch, install Gatsby CLI, and create a new project:
$ npm install -g gatsby-cli
$ gatsby new gatsby-example
We can now see a gatsby-example directory with all the assets you need to develop your Gatsby site.
Navigate to your directory and add gatsby to the dependencies of your package.json file by running:
$ npm install gatsby-cli --save
Compile your site by running:
$ gatsby develop
You can see the Gatsby starter site at http://localhost:8000/:
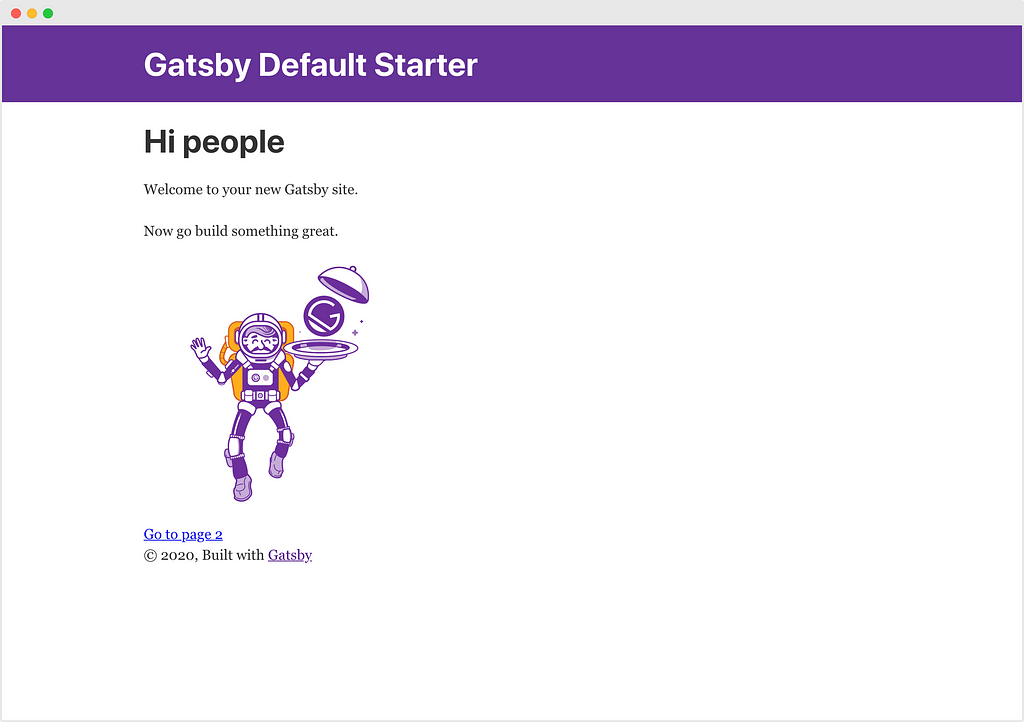
Now we’re ready to add Percy for visual testing. 👀
Installing Percy’s Gatsby plugin
Percy’s Gatsby plugin is powered by Percy CLI and works by taking screenshots every time you build your site. By default, it takes snapshots of your /public directory, but you can configure it to match your setup or to ignore certain pages.
To get started, navigate to your directory and run:
$ npm install --save @percy/agent gatsby-plugin-percy
This may take a few minutes as we download all the dependencies needed to capture the DOM state of your pages and render screenshots. Once it’s finished installing, open your gatsby-config.js file and add 'gatsby-plugin-percy' to the list of plugins. It should look something like this:
module.exports = {
siteMetadata: {
title: `Gatsby Default Starter`,
description: `Kick off your next, great Gatsby project with this default starter. This barebones starter ships with the main Gatsby configuration files you might need.`,
author: `@gatsbyjs`,
},
plugins: [
`gatsby-plugin-react-helmet`,
{
resolve: `gatsby-source-filesystem`,
options: {
name: `images`,
path: `${__dirname}/src/images`,
},
},
`gatsby-transformer-sharp`,
`gatsby-plugin-sharp`,
`gatsby-plugin-percy`,
{
resolve: `gatsby-plugin-manifest`,
options: {
name: `gatsby-starter-default`,
short_name: `starter`,
start_url: `/`,
background_color: `#663399`,
theme_color: `#663399`,
display: `minimal-ui`,
icon: `src/images/gatsby-icon.png`, // This path is relative to the root of the site.
},
},
// this (optional) plugin enables Progressive Web App + Offline functionality
// To learn more, visit: https://gatsby.dev/offline
// `gatsby-plugin-offline`,
],
}
Let’s run our first build locally to make sure everything is set up correctly.
Running your first Percy build
If you haven’t already, sign up for a free Percy account. Percy’s free plan includes unlimited projects, 10 users, and 5,000 snapshots each month.
Name your organization and create your first project. Percy projects correspond with the apps, sites, or component libraries you want to test.
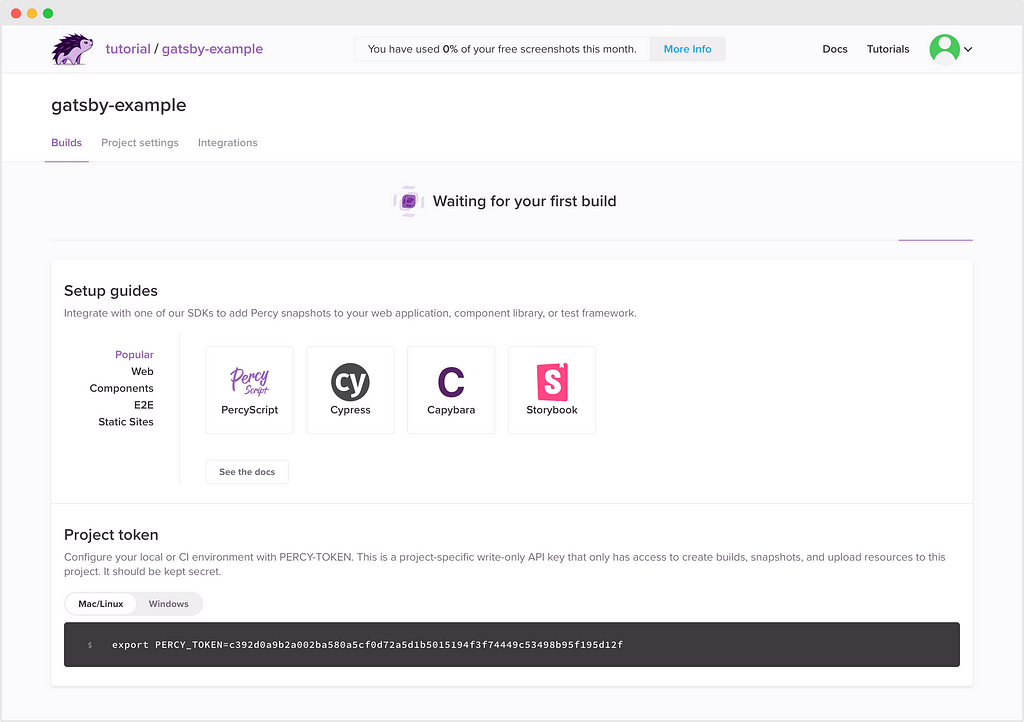
Each has a project-specific API token that we’ll use to configure our local environment now, and our Netlify environment later.
Copy and set your PERCY_TOKEN in your local environment and run your first build:
# If you're using Windows:
$ set PERCY_TOKEN=<your token here>
# Otherwise:
$ export PERCY_TOKEN=<your token here>
$ gatsby build
This will compile your site, call the Percy plugin, and trigger Percy to render screenshots of all the pages located in your project’s /public directory.
Click the link in your terminal output to check out your screenshots:
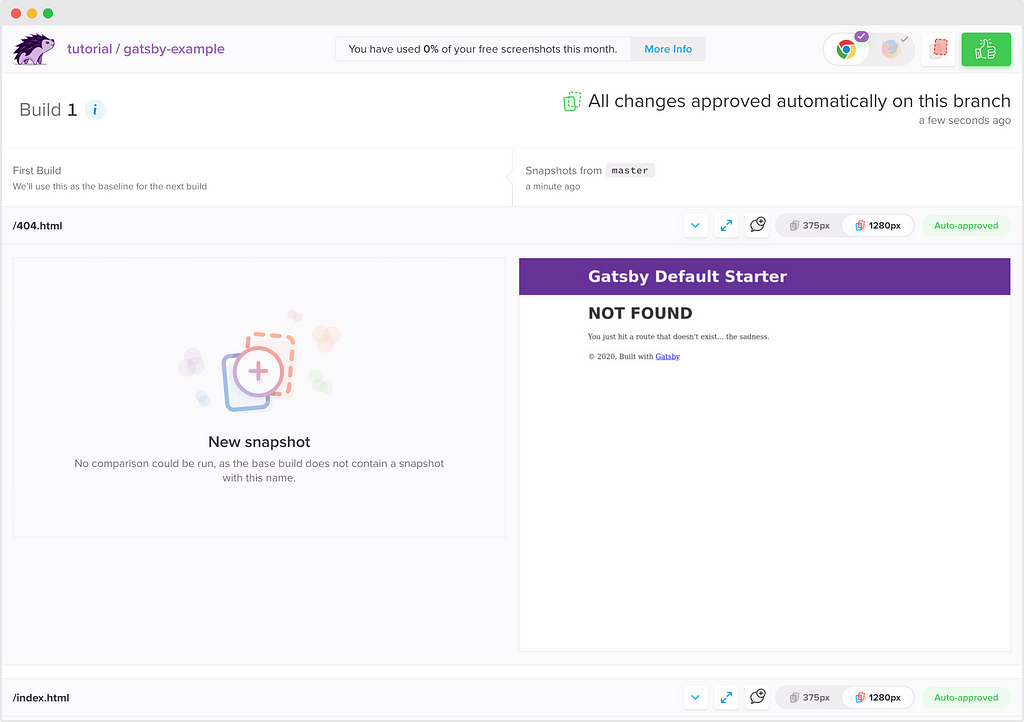
Notice that because this is your first build, there’s nothing to compare the snapshots to. The snapshots in this build will be the baselines for snapshots in subsequent builds.
Now that we’ve run our first build locally, let’s get our code into GitHub and get ready to connect Percy and Netlify.
Setting up your GitHub repo
Start by making a new GitHub repository for your example app.
After navigating to your directory, initialize it as a Git repo, add the files in your local repo, commit the files you’ve staged, and push them to your new repository.
$ git init
$ git add .
$ git commit -m ‘First commit'
$ git remote add origin: https://github.com/[name]/[repository].git
$ git push -u origin master
Now that your code is on GitHub, head to your Percy project, select “Add integration,” and follow all the steps to install the GitHub integration. Once you’ve authorized GitHub, you need to link your Percy project with your GitHub repo.
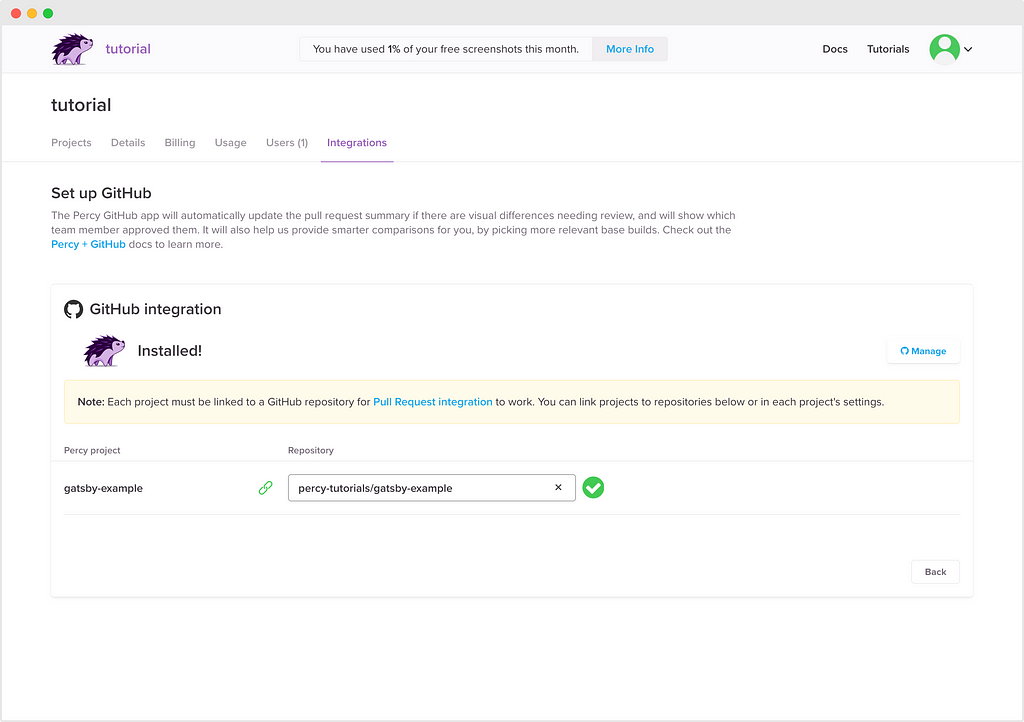
After you’ve installed Percy’s GitHub integration, your visual testing results and alerts will appear in your commits and pull request checks, making it easy to review changes. A pull request integration also provides Percy with better metadata when new builds are created and can help in selecting the most accurate baseline for pull request builds.
Getting continuous deployments with Netlify
Sign up for a Netlify account and select “New site from Git.” Once you’ve authorized Netlify and linked your example site repository, configure your settings and hit “Deploy site.” Alternatively, you can deploy directly from the README.md in your Gatsby starter GitHub repo. Either way, Netlify will immediately start deploying your site.
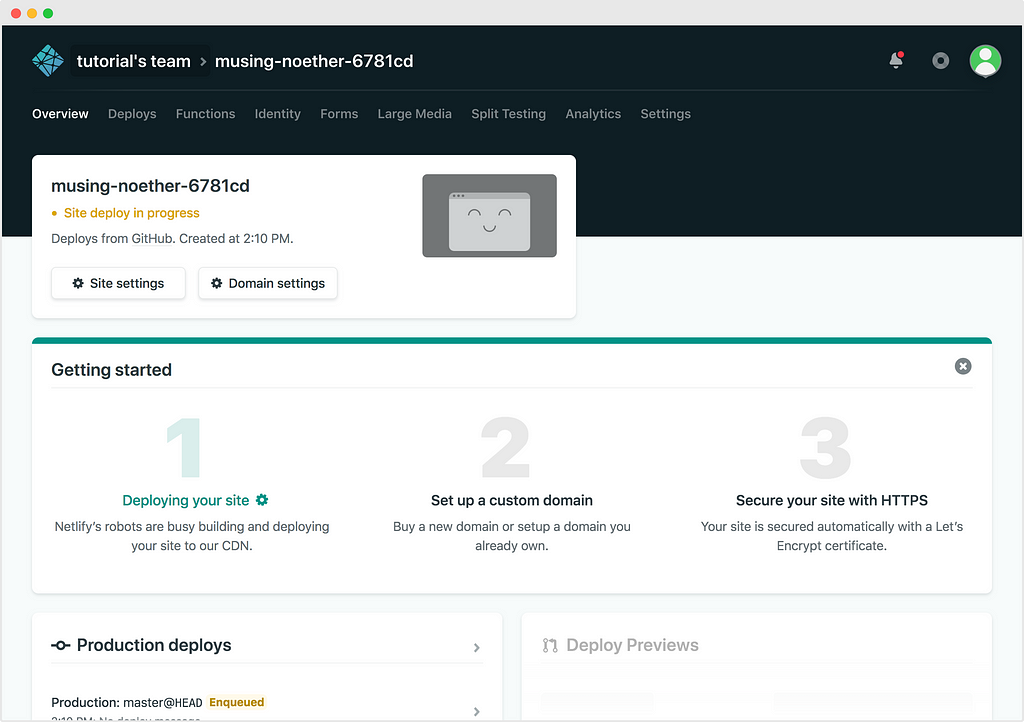
Next, let’s connect Netlify with our Percy project. Navigate to your Netlify dashboard, go to Settings > Build & deploy > Environment > Environment variables. Paste your PERCY_TOKEN, the API key we used earlier; you can find it in your Project settings.
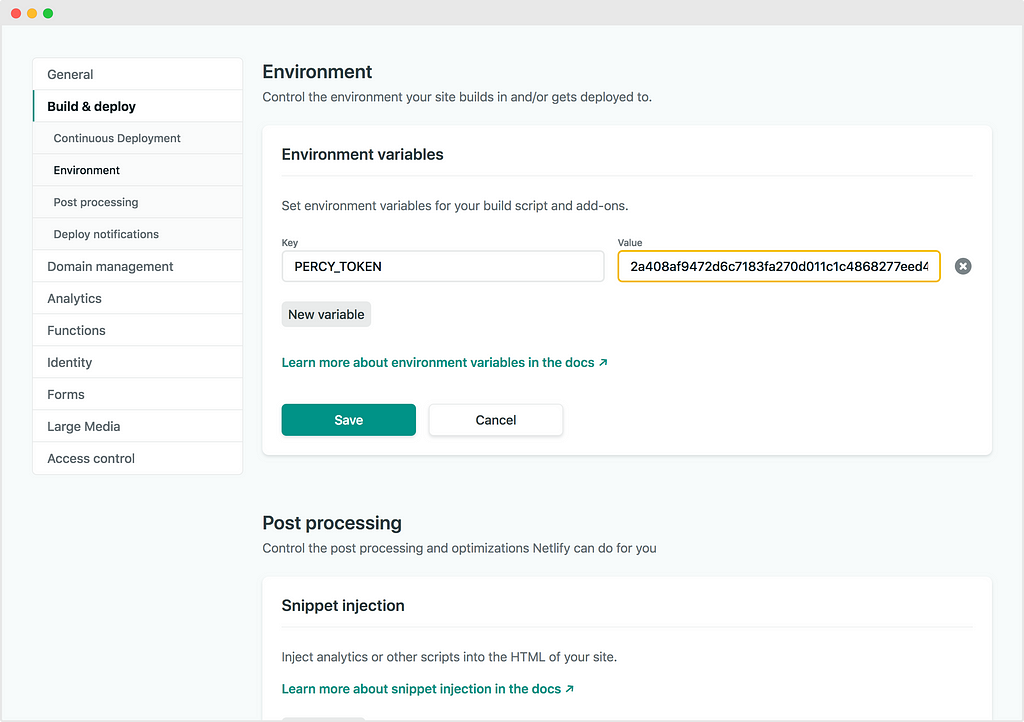
Now that we’ve integrated everything, we’re ready to do our first end-to-end visual review.
End-to-end visual review
Let’s make a code change that will result in visual changes to our site, build our site, and review the changes in Percy.
For simplicity’s state, we’re going to make a small text change to our index.js file, changing line 11 in honor of our mascot 💜:
import React from "react"
import { Link } from "gatsby"
import Layout from "../components/layout"
import Image from "../components/image"
import SEO from "../components/seo"
const IndexPage = () => (
<Layout>
<SEO title="Home" />
<h1>Hi porcupines</h1>
<p>Welcome to your new Gatsby site.</p>
<p>Now go build something great.</p>
<div style=>
<Image />
</div>
<Link to="/page-2/">Go to page 2</Link>
</Layout>
)
export default IndexPage
Commit those changes to a new branch and watch Netlify spin up a deploy preview, complete its various checks, and trigger Percy. Percy will render new screenshots for your compiled site and compare them against the snapshots from our first build.
Since there are visual changes, Percy will show up in your pull request as needing review:
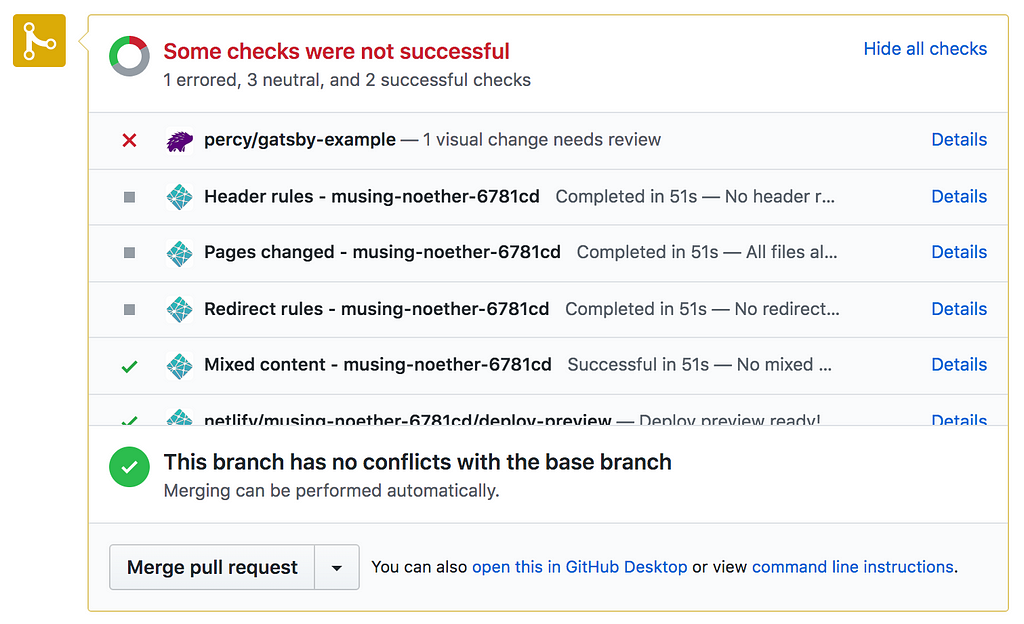
Clicking “Details” will take you to the Percy UI where you can review the visual change:
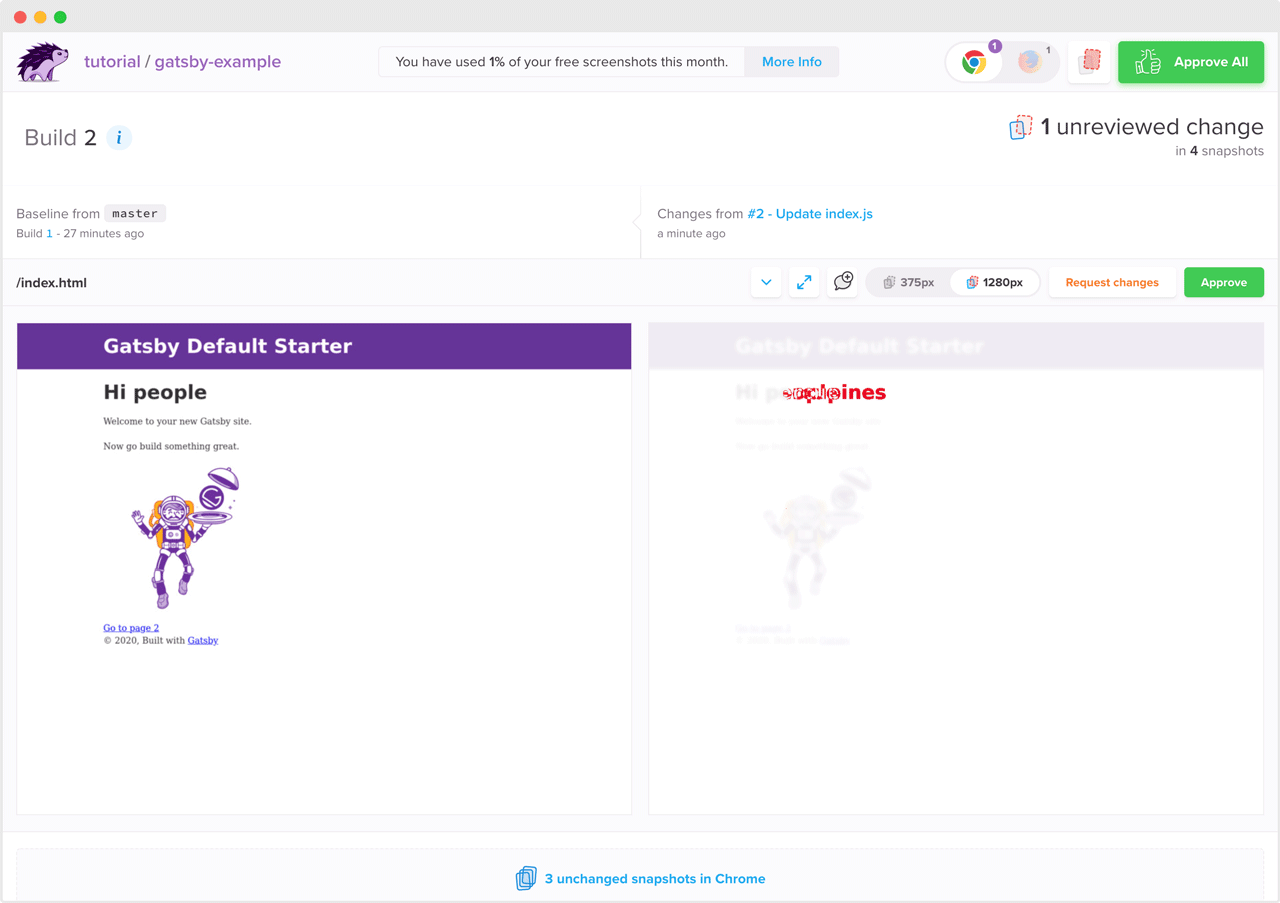
The red highlighting in the right panel represents the part of your page that has changed. Clicking that area (or pressing “d”) will toggle between the diff and the underlying screenshot so you can easily see what has changed.
Your pages have been rendered across different responsive widths and browsers to help detect regressions across those different environments.
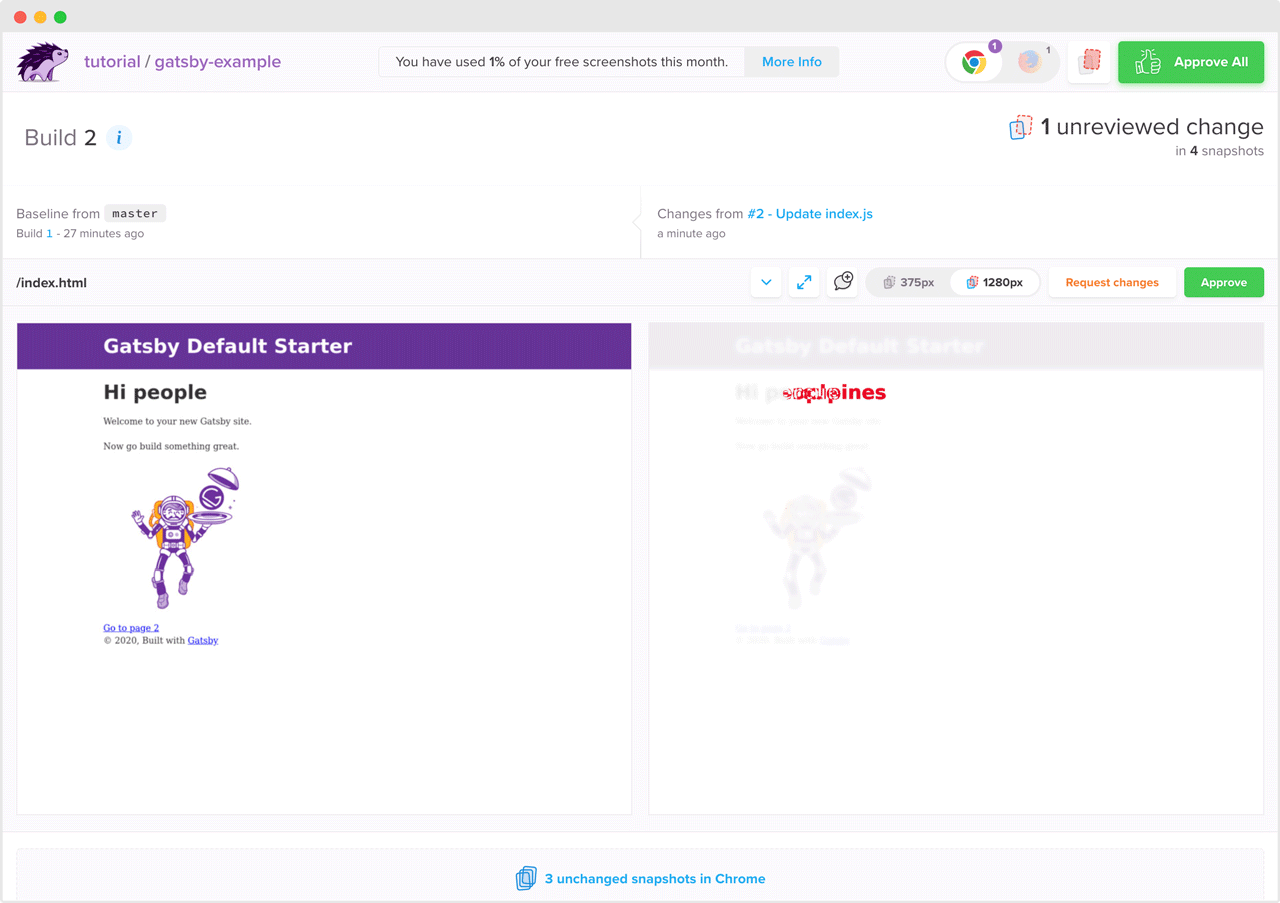
By default, Percy renders across Chrome and Firefox, both at 375px and 1280px. To configure custom widths, check out our global @percy/agent config docs.
Reviewing visual changes
With Percy, you can either Approve ✅ or Request changes 🙅♀️as well as leave comments 💬 on snapshots.
If you’re happy with your changes, you can approve individual snapshots or “Approve all” to signify that everything looks good. Alternatively, if the detected changes were unintentional or otherwise are not ready to be merged, marking them as “Changes requested” will block the build from being approved. This feature, coupled with snapshot comments, makes it easy for teams to collaborate and stay on the same page throughout visual reviews.
Since we meant to make this change, let’s hit “Approve.” Heading back over to our pull request, we’ll see that all our pull request check is green:
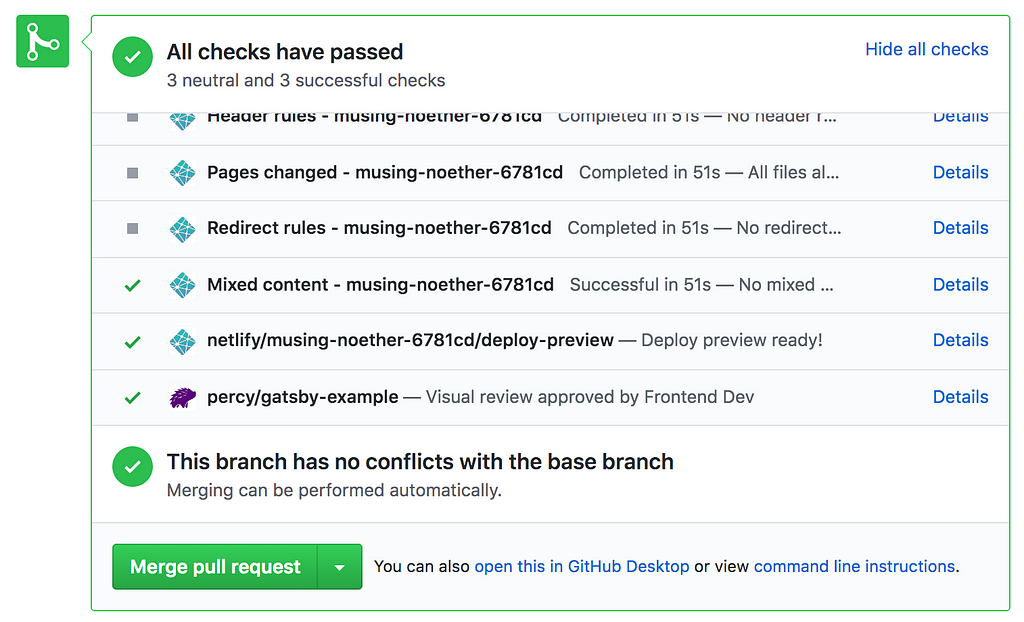
Congratulations on doing your first visual review! ✨
We hope this tutorial helped you get started with Percy for Gatsby and Netlify and gave you a glimpse into the powers fo visual testing.
We’re thrilled to make it easier to integrate Percy with Gatsby and to provide first-class support for Netlify. If you have questions or need help, don’t hesitate to reach out to support via our website chat or by emailing support@percy.io.
<hr><p>Tutorial: Visual testing for Gatsby and Netlify with Percy was originally published in Percy Blog on Medium, where people are continuing the conversation by highlighting and responding to this story.</p>